when(myClass.calculateY(1)).thenReturn(2);It will give me the error: exceptions.misusing.MissingMethodInvocationException: when() requires an argument which has to be 'a method call on a mock'.
Solution:
use @Spy tag like this:
@InjectMocks
@Spy
private MyClass myClass;
An important difference between the Spy and Mock classes is that we previously saw that there are 2 ways to add behavior to a Mock.
In Mock’s case, the actual method is never called.
But in the case of @Spy there is an important difference here, since in one case the real method is NOT called and in the other it is. Let’s see an example.
In this case the real method is NOT invoked:
Mockito.doReturn(false).when(spyClass).isActive();
In this case, the real method will be invoked:
Mockito.when(spyClass.isActive()).thenReturn(false);
Provide argument matcher
Sometimes maybe we just don’t care about the actual value being passed as an argument, so in those cases we can use some of the following argument matchers:
anyString(), anyInt(), anyBoolean(), any(), any(Date.class)
So in our previous example instead of passing the value 1 to the calculateY method we can do the following:
when(service.calculateY(Mockito.anyInt())).thenReturn(2);
Something to keep in mind here is that it cannot be mixed with real values.
For example this line of code:
Mockito.verify(ServiceName, times(1)).functionName("test@email.com", any());
If we run it as JUnit test in Eclipse, we can see that the Junit view Console shows us the following error message:
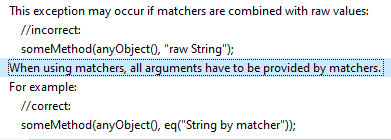
Solution:
Mockito.verify(ServiceName, times(1)).functionName(Mockito.eq("test@email.com"),any());
Or
Mockito.verify(ServiceName, times(1)).functionName("test@email.com","real name");
Another aspect that we can notice here is that Mockito shows a good error message including even possible solutions. So pay attention to the error messages.
So as we have seen, Mockito allows us to easily isolate our classes so that we can test them without worrying about the other modules.
The only thing left is to generate your test code, Mock what you need to Mock, invoke the method and verify that the result is what you expected!